In this article, I will explain to you in detail along with java code to find the nth element in the array in java.
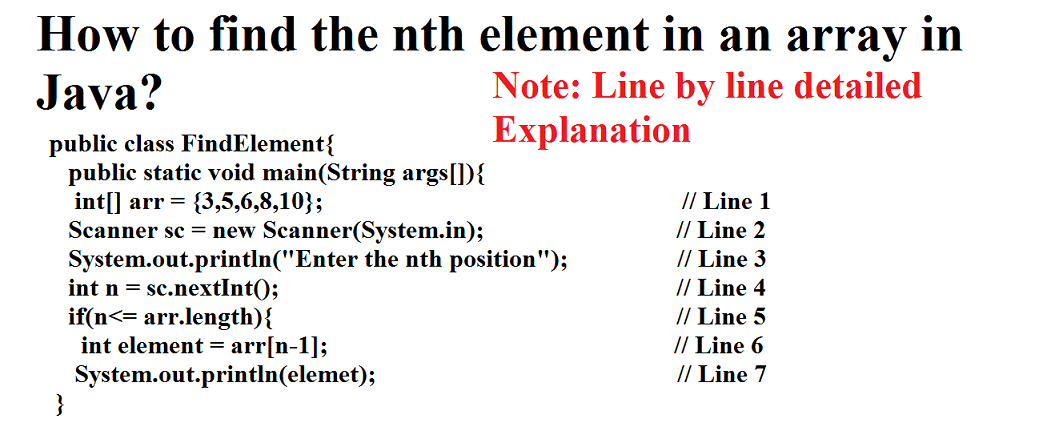
public class FindElement{
public static void main(String args[]){
int[] arr = {3,5,6,8,10}; // Line 1
Scanner sc = new Scanner(System.in); // Line 2
System.out.println("Enter the nth position"); // Line 3
int n = sc.nextInt(); // Line 4
if(n<= arr.length){ // Line 5
int element = arr[n-1]; // Line 6
System.out.println(elemet); // Line 7
}
else {
System.out.println("Enter any position upto" + arr.length) //Line 8
}
}
Explanation of Each Line
Line 1 : int[] arr = {3,5,6,8,10};
In this line, I declared one array in variable arr and initialize with 5 elements. So the total length or size of this array is 5.
Line 2 : Scanner sc = new Scanner(System.in);
In this line Scanner class object is created. The purpose of this object is to take input from the end-user or keyboard. Scanner class contains many methods or function which has been written by the java team. Using that method we can take input value from the end-users.
Line 3 : System.out.println(“Enter the nth position”);
In this line, println() method will show the message in the screen of the enter users, who want to know the nth position element in the array.
Line 4 : int n = sc.nextInt();
Here once the user input some number it will store in the variable with name “n”. Suppose the end-user wants to know the element at position 4, So the n =4.
sc.nextInt():- Here “sc” is an Object Reference of Scanner Class, which represents or refers to the Object of Scanner class. We are calling a method named “nextInt” on object reference of Scanner class. This method helps us to take the input of datatype int from the user.
Note: Scanner class contains many methods such as next(), nextLine(), etc. so depending upon what is the datatype of the number which the end-user will input from their keyboard. If they will input the decimal number like 4.50 then you need to write sc.nextFloat(); But for our case, the nth position should always be an integer.
Line 5 : if(n<= arr.length)
This line is checking whether the position enters by the user is less than the size or length of the array, if it is more than the length of the array then that time the mentioned position by the user does not exist.
Suppose the user enter wants to know the 6th position element in the array , but we clearly see we have 5 elements in total, it means the maximum position of our array is 5. Hence, in this case, we will display in the user’s to enter the position within the range of 5 in this case.
Line 6 : int element = arr[n-1];
Here we are getting the nth postion element and storing in the variable named element. Suppose , user want to know 4th position element then n = 4. And we all know the array is index based that means index always starts with 0. So , the element present in at first position has index value is 0.
arr[n-1] : Here inside the square bracket “[]” we give the index value . So If users want to know the element at position 4, then the index must be 3 inside the square bracket.
arr[4-1] = arr[3] = 8 , So the element present in at 4th postion is 8 in this array arr = {3,5,6,8,10}. Hence the answer is 8 , if user enter 4.
Line 7 : System.out.println(elemet);
This line will print the nth postion element on the screen of end user.
Line 8 : System.out.println(“Enter any position upto” + arr.length)
This is else part code, if the user enters the position number more than size or length of array then, the above message will display on his screen, which indicates that he can enter position should be less or equal to the size of the array.
arr.length: It will display the length of array. For our case, we have 5 elements so arr.length = 5.
Thanks a lot for reading my article. If I tried to explain each line in detail, but you still have any doubts or queries, please feel free to comment down below. I will love to solve your queries.
Also check out my other articles of Java OOPs concepts such as Inheritance, Encapsulation, Abstraction, etc.