In this article, we will develop a Spring Boot Web Application using Thymeleaf. Basically it a modern server-side engine for java templates. It supports templates such as HTML5, XML, and many more.
What is Thymeleaf?
Thymeleaf is a java template engine which used for creating a web as well as a standalone application. Since it is a java based so, you can also consider it a Java-based library.
It supports many types of templates such as
- HTML5
- Legacy HTML5
- XML
- XHTML
- Valid XML
To implement Thymeleaf in our spring boot application we required following dependency.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
Let us begin to create a Spring Boot Web Application using Thymeleaf, just follow the below steps and you will able to build your web application. At the last, I will give the link to download the Source code of this project.
Step 1: Go to Spring Initializr and create a Maven Project.
Step 2: Give the group name . I have given com.pixeltrice.
Step 3: Enter a artifact id. spring-boot-thymeleaf-application.
Step 4: Add the dependencies such as Spring Web and Thymeleaf.
Step 5: Click on the Generate button. Once we click, our project will start downloading on the local system in the form of a zip file.
Step 6: Unzipped it and import into your IDE workspace. I am using Eclipse.
Select File -> Import -> Existing Maven Projects -> Browse -> Select the folder spring-boot-thymeleaf-application-> Finish.
Step 7: Create a controller class for HTTP Endpoints or API as shown below.
package com.pixeltrice.springbootthymeleafapplication;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class ThymeleafController {
@RequestMapping(value ="/home")
public String index() {
return "index";
}
}
In the above code, when someone hits or calls the API localhost:8080/home it will redirect to the HTML page named index.html.
Step 8: Create a file styles.css in the following path src/main/resources/static/css/
h4 {
color: green;
font-size: 57px;
}
Note: All the styles and javascript related files should always be placed in the path src/main/resources/static or under static folder.
Step 9: Create the index.html under following path src/main/resources/templates
<!DOCTYPE html>
<html>
<head>
<meta charset = "ISO-8859-1" />
<link href = "css/styles.css" rel = "stylesheet"/>
<title>Spring Boot Web Application</title>
</head>
<body>
<h4>Welcome to Spring Boot Thymeleaf web application</h4>
</body>
</html>
Step 10: Make sure that pom.xml must have following dependency.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
So, the final pom.xml file should be look like as shown below.
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.3.2.RELEASE</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>com.pixeltrice</groupId>
<artifactId>spring-boot-thymeleaf-application</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>spring-boot-thymeleaf-application</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>11</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
<exclusions>
<exclusion>
<groupId>org.junit.vintage</groupId>
<artifactId>junit-vintage-engine</artifactId>
</exclusion>
</exclusions>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
Step 11: SpringBootApplication or main class should be look like as shown.
package com.pixeltrice.springbootthymeleafapplication;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class SpringBootThymeleafApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootThymeleafApplication.class, args);
}
}
Final Project Explorer folder structure.
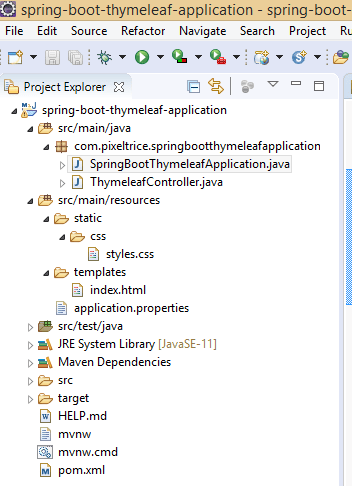
Step 12: Run the Spring boot Application and when you hit the following endpoint or URL from browser localhost:8080/home, you will get the screen as shown below.

Summary
Thank you so much for reading this article. In this article, we learned to create a Spring Boot Thymeleaf web application. You can do lots of staff such as add HTML tags for the table, or implement the Login form page in that HTML and many more. If you have any queries or doubt please do comment below or you can send me in my email from Contact Us page.
You can also checkout my other articles on Spring Boot.
- Spring Boot Security using OAuth2 with JWT
- Deploy the Spring Boot Application on External Tomcat Server
- How to send email using Spring Boot Application
- How to add an Interceptor in a Spring Boot Application
- Run Application from Spring Boot CLI