Map interface in Java is a collection of entries that means you can store multiple entries on the map.
Entry
An entry is data in the form of a key-value pair wherein key must always be unique but the value can be the same.
Note: The value of entry can be different, same or null, it can be anything, but the key must always be unique and it should not be null.
Syntax of Map
Map<key, Value>
When we use Map in Java?
If the requirement is to store the multiple data in the form of a key-value pair then we go for a map. Technically, when we want to represents a group of objects in the form of key-value pairs then we go for Map.
Real-World Example
Suppose I want to store details of some particular person, such as his first name, last name, address, and gender.
Step 1: Create an Object of the Class which is the child of Map Interface.
Map<String, String> personDetails = new HashMap<>();
Explanation:
Left side of assignment operator (=)
We have Map<String, String> inside this angular bracket <> the first one represents the datatype of key and the second one represents the datatype of value.
Since in the above example, the keys are the first name, last name, address, and gender and all are String. So the datatype of keys is String that’ s why in the angular bracket it is mentioned String.
Now lets come to value part, we are going to assign some value in the first name, last name, address and in gender, we all know these data also have a type of String. Hence in second place in the angular bracket, it is mentioned, String.
personDetails is an Object Reference of Object HashMap<>();
Right side of the assignment operator (=)
On the Right side, we have “new HashMap<>()”. Basically we have created an Object of HashMap class. Since Map is an interface and we know in java we cannot directly create the object of the interface.
There must be at least one class that implements the interface to create Object of it. So here HashMap is one of the implementation classes of the Map interface.
Step 2: Adding the data inside the Object.
For adding the data inside the Map Object we have to use one inbuild method present in Java that is put().
personDetails.put(“firstName”,”John”); —–> First Entry in Map.
personDetails.put(“lastName”, “Cena”); ——-> Second Entry in Map.
personDetails.put(“address”, “New York , America”); ——–> Third Entry in Map.
personDetails.put(“gender”,”male”); ——–> Forth Entry in Map.
Now you map has all necessary data in the form of entries. We have in total 4 entries in above example.
Step 3: Print the data present inside the Map.
System.out.print(personDetails);
Output
{ firstName = John, lastName = Cena, address = New York, America, gender = male }
Code in Java
import java.util.*;
public class Main{
public static void main(String args[]){
Map<String, String> personDetails = new HashMap<>();
personDetails.put("firstName","John");
personDetails.put("lastName", "Cena");
personDetails.put("address", "New York , America");
personDetails.put("gender","male");
System.out.print(personDetails);
}
}
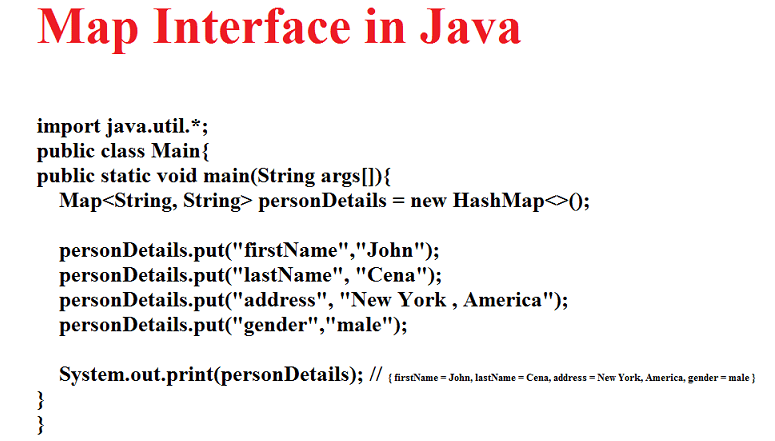
Methods Present in Map Interface
Return Type | Method | Description |
void | clear() | It will remove all the elements present in the map. Example: personDetails.clear(); All the entries will get removed from map. System.out.print(personDetails); Output : { } |
boolean | containsKey(Object key) | Used to check that a particular key is present on the map or not. Example : personDetails.containsKey(“firstName”)”) Output: true. |
boolean | containsValue(Object value) | Check inside the map whether any of the key having a particular value or not. Example: personDetails.containsValue(“John”); Output : true. |
valueDataType | get(Object key) | It returns the value for a particular key. Example : personDetails.get(“firstName”); Output: John |
boolean | isEmpty() | Check whether the map is empty or having some entry. |
void | put(Key, Value) | Used to add an entry or key-value pairs inside the map. As you can see in the above section I explained it in detail. |
void | putAll(Map m) | It used to add entries from existing map into another map. Example: Map<String, String> anotherMap = new HashMap<>(); anotherMap.put(“location”,”London”); personDetails.putAll(anotherMap); Output: { firstName = John, lastName = Cena, address = New York, America, gender = male , location = London} |
void | remove(Object key) | It will remove the particular entry of the key which we pass as an argument. |
int | size() | It provides the number of key-value pairs or entry present inside the Map. |
Set<Key> | keySet() | It returns the all keys present in the Map. |
Collection<Values> | values() | It returns all the values present in the map. |
Set<Map.Entry<>Key, Value> | entrySet() | It returns the map in the form of a Set. Example: we have personDetails as { firstName = John, lastName = Cena, address = New York, America, gender = male } Now, with entrySet() we will get personDetails.entrySet(); Output : [ firstName = John, lastName = Cena, address = New York, America, gender = male ] Have noticed changes in braces from {} to []. |
Implementation Classes of Map Interface
Following are classes which implements the Map Inteface.
- HashMap
- TreeMap
- Hashtable
Thank You so much for reading this article. I hope you learn something new from here. You can also check out my other articles such as Inheritance, Abstraction, etc.
If you have any queries or suggestion please feel free to ask. I will respond you as soon as possible.